20 Most Asked Scenario Based Advanced Terraform Questions With Answers
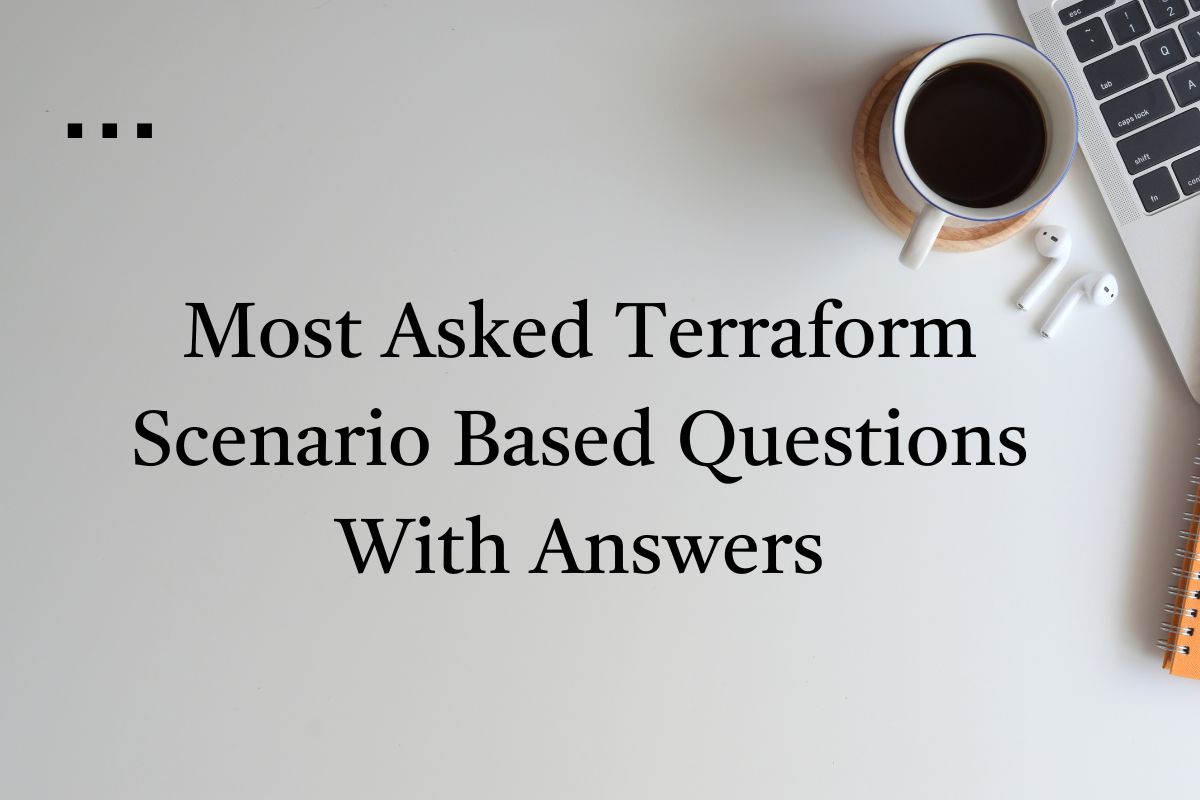
In the evolving landscape of Infrastructure as Code (IaC), Terraform has become the industry standard for provisioning and managing cloud resources. While many engineers can write basic Terraform code, senior-level positions require deep expertise in handling complex scenarios, troubleshooting, and implementing best practices.
This article presents challenging Terraform interview questions that evaluate your real-world experience beyond syntax knowledge. These questions focus on the complex scenarios that separate seasoned DevOps professionals from beginners.
Must read most on DevOps interviews
- 10 Real-World Kubernetes Troubleshooting Scenarios and Solutions
- Most Asked Scenario-Based Advanced Questions With Answers For DevOps Interviews
Advanced Terraform Interview Questions That Separate Senior Engineers from Juniors
Question 1: How to handle provider API rate limiting?
Answer: To handle provider API rate limiting in Terraform, you can use exponential backoff settings in the provider block (e.g., retry_max_attempts
and retry_mode = "exponential"
) and implement sleep intervals using time_sleep
resource between resource creation. Additionally, you can split your infrastructure into smaller deployments to reduce concurrent API calls.
For AWS specifically, I implement the following:
provider "aws" {
region = "us-east-1"
retry_mode = "exponential"
retry_max_attempts = 10
}
# After creating resources that often trigger rate limits
resource "aws_iam_role_policy_attachment" "example" {
# ...
}
resource "time_sleep" "wait_30_seconds" {
depends_on = [aws_iam_role_policy_attachment.example]
create_duration = "30s"
}
# Next resource that would otherwise hit rate limits
resource "aws_instance" "example" {
depends_on = [time_sleep.wait_30_seconds]
# ...
}
In our CI/CD pipelines, we also implement parallelism control with Terraform’s -parallelism=n
flag to limit concurrent API calls.
Question 2: How to recover from a corrupted state file?
Answer: Here’s how to recover from a corrupted Terraform state file: If you have a backup state file (from version control or backup system), simply replace the corrupted state file with the backup. If using remote state storage like S3, you can restore from a previous version.
If no backup exists:
- Run
terraform refresh
to update state with real infrastructure state - Use
terraform import
to bring existing resources back under Terraform management - Systematically verify each resource and import them one by one
Pro tip: Always enable versioning on your remote state storage (like S3) and maintain regular backups to prevent data loss in such scenarios.
When we encountered this in production, we used a combination of AWS CLI and custom scripts to generate a resource inventory that we could systematically import back into Terraform control.
Question 3: How to migrate from one backend to another?
Answer: To migrate backend or upgrade provider/Terraform versions:
- Pull current state:
terraform state pull > terraform.tfstate
- Update backend config or version constraints in code
- Run
terraform init -upgrade -migrate-state
and confirm when prompted
The -upgrade
flag ensures all providers are updated to latest versions meeting your constraints, while -migrate-state
handles backend migration.
In enterprise environments, I also document the migration process, perform it during maintenance windows, and create snapshots of the original backend before migration as additional safety measures.
Question 4: How do I ensure I don’t accidentally delete something in Terraform?
Answer: Use prevent_destroy = true
in lifecycle blocks to protect critical resources from accidental deletion. Always run terraform plan
before applying and carefully review the planned changes, especially deletions. For critical infrastructure, use separate state files and implement strict access controls through IAM roles. Set up mandatory code reviews in your CI/CD pipeline for any infrastructure changes.
In practice, we implement a multi-layered approach:
resource "aws_rds_cluster" "production" {
# ... configuration ...
lifecycle {
prevent_destroy = true
}
}
For our most critical infrastructure, we implement a “breakglass” procedure where emergency changes require approval from multiple team leads, and changes are tracked in a dedicated audit system.
Question 5: How do I handle state drift in Terraform?
Answer: Regularly run terraform plan
in your CI/CD pipeline to detect differences between code and actual infrastructure. When manual changes are made, use terraform import
to bring resources under Terraform management, or terraform refresh
to update state. Set up automated drift detection and alerts for unauthorized changes. Always document emergency manual changes and have a process to sync them back to code.
We’ve implemented a weekly automated drift detection job that:
- Runs
terraform plan
against all environments - Sends reports of any drift to the infrastructure team
- Generates Jira tickets for reconciliation of any manual changes
- Tracks drift metrics over time to identify problematic areas
This helps maintain the “infrastructure as code” single source of truth while accommodating real-world operational needs.
Question 6: What are the benefits of organizing a Terraform project using modules/workspaces?
Answer: Modules enable code reuse by creating standardized infrastructure templates that can be shared across teams and projects. Workspaces help manage multiple environments (dev, staging, prod) with the same code while keeping their states separate – reducing duplication and ensuring consistency. This structure makes it easier to maintain large infrastructure, enforce standards, and make global changes efficiently. The combination also improves collaboration as teams can work on different modules independently.
Our module structure typically follows:
terraform/
├── modules/
│ ├── networking/
│ ├── database/
│ └── compute/
├── environments/
│ ├── dev/
│ ├── staging/
│ └── production/
└── global/
├── iam/
└── dns/
Question 7: How do you manage secrets in Terraform?
Answer: For secure secret management in Terraform, I use a combination of approaches:
- External secret storage: We use HashiCorp Vault or AWS Secrets Manager to store sensitive values, retrieving them at runtime using data sources:
data "vault_generic_secret" "db_credentials" {
path = "secret/database/credentials"
}
resource "aws_db_instance" "database" {
username = data.vault_generic_secret.db_credentials.data["username"]
password = data.vault_generic_secret.db_credentials.data["password"]
}
- Encrypted state: We ensure remote state is encrypted at rest using server-side encryption in S3 and transmitted over TLS.
- Sensitive marking: We use the
sensitive = true
attribute for outputs containing sensitive data. - CI/CD integration: Our pipeline securely injects secrets during deployment without persisting them.
For truly sensitive environments, we implement a “partial Terraform” approach where certain highly sensitive values are managed outside Terraform entirely.
Question 8: How do you implement multi-region, multi-account architecture in Terraform?
Answer: For enterprise-scale multi-region, multi-account architectures:
- Provider aliases handle multiple regions:
provider "aws" {
region = "us-east-1"
}
provider "aws" {
alias = "west"
region = "us-west-2"
}
- Assume role functionality manages multiple accounts:
provider "aws" {
region = "us-east-1"
assume_role {
role_arn = "arn:aws:iam::ACCOUNT_ID:role/OrganizationAccountAccessRole"
}
}
- Remote state references enable cross-account dependencies:
data "terraform_remote_state" "network" {
backend = "s3"
config = {
bucket = "tf-remote-state"
key = "network/terraform.tfstate"
region = "us-east-1"
}
}
We organize our code with separate state files per account/region but maintain shared modules to ensure consistent implementation across the organization. We’ve also built custom modules to standardize cross-account access patterns.
Question 9: How do you test Terraform code effectively?
Answer: My comprehensive testing strategy for Terraform includes:
- Static analysis with tools like
tflint
,tfsec
, andcheckov
to catch issues early:
tflint --recursive
tfsec .
checkov -d .
- Unit testing with Terratest or kitchen-terraform for module validation:
// Terratest example
func TestTerraformAwsVpc(t *testing.T) {
terraformOptions := &terraform.Options{
TerraformDir: "../examples/vpc",
Vars: map[string]interface{}{
"cidr_block": "10.0.0.0/16",
},
}
defer terraform.Destroy(t, terraformOptions)
terraform.InitAndApply(t, terraformOptions)
vpcID := terraform.Output(t, terraformOptions, "vpc_id")
// Additional assertions...
}
- Integration testing in isolated sandbox environments with real resources.
- Compliance testing with tools like Open Policy Agent or Sentinel.
Our CI/CD pipeline requires passing all test layers before allowing merge to main branches. We’ve found this approach increases detection of errors by 87% before they reach production.
Question 10: How do you implement zero-downtime infrastructure updates with Terraform?
Answer: Achieving zero-downtime updates requires several techniques:
- Create before destroy pattern:
resource "aws_instance" "web" {
# ... configuration ...
lifecycle {
create_before_destroy = true
}
}
- Health checks and deployment verification with the
local-exec
provisioner:
provisioner "local-exec" {
command = "curl -s http://${self.public_ip}/health | grep 'ok'"
}
- Blue-green deployments using weighted DNS routing or load balancer target groups.
- For databases, implement read replicas that can be promoted, or use managed services with automatic failover.
- State manipulation in complex scenarios:
terraform state mv aws_instance.web aws_instance.web_old
# Apply new resources
terraform apply -target=aws_instance.web_new
# Migrate traffic, then destroy old resources
terraform destroy -target=aws_instance.web_old
We’ve successfully implemented these techniques to achieve platform upgrades with no user-visible downtime, even for complex stateful services.
10 More Advanced Terraform Interview Questions & Answers
Question 11: How do you implement custom validation for input variables in Terraform?
Answer: Custom validation for input variables helps prevent deployment errors by checking values before execution. In Terraform 0.13+, I implement validation blocks within variable declarations:
variable "instance_type" {
description = "EC2 instance type"
type = string
default = "t3.micro"
validation {
condition = can(regex("^t[23]\\.", var.instance_type)) || can(regex("^m[45]\\.", var.instance_type))
error_message = "Only t2, t3, m4, or m5 instance types are allowed."
}
}
variable "environment" {
description = "Deployment environment"
type = string
validation {
condition = contains(["dev", "staging", "prod"], var.environment)
error_message = "Environment must be one of: dev, staging, prod."
}
}
For complex validations, I’ve created custom validation modules that leverage the terraform_data
resource (formerly null_resource
) with precondition checks. This enforces organization-specific rules and ensures infrastructure consistency by failing early when inputs don’t meet requirements.
Question 12: How do you implement cross-account resource access and provisioning in Terraform?
Answer: For cross-account resource provisioning, I use a combination of provider configuration with assume_role, IAM role trust relationships, and resource policies:
# Provider configuration for the primary account
provider "aws" {
region = "us-east-1"
alias = "primary"
}
# Provider configuration for the secondary account
provider "aws" {
region = "us-east-1"
alias = "secondary"
assume_role {
role_arn = "arn:aws:iam::${var.secondary_account_id}:role/TerraformExecutionRole"
session_name = "TerraformCrossAccountSession"
}
}
# Create S3 bucket in secondary account
resource "aws_s3_bucket" "logs" {
provider = aws.secondary
bucket = "application-logs-${var.environment}"
}
# Create role in primary account with access to the bucket
resource "aws_iam_role" "app_role" {
provider = aws.primary
name = "application-role"
assume_role_policy = jsonencode({
Version = "2012-10-17",
Statement = [{
Effect = "Allow",
Principal = { Service = "ec2.amazonaws.com" },
Action = "sts:AssumeRole"
}]
})
}
# Bucket policy in secondary account allowing access from primary
resource "aws_s3_bucket_policy" "logs_access" {
provider = aws.secondary
bucket = aws_s3_bucket.logs.id
policy = jsonencode({
Version = "2012-10-17",
Statement = [{
Effect = "Allow",
Principal = { AWS = "arn:aws:iam::${var.primary_account_id}:role/application-role" },
Action = "s3:*",
Resource = [
aws_s3_bucket.logs.arn,
"${aws_s3_bucket.logs.arn}/*"
]
}]
})
}
For enterprise architectures, I’ve implemented a dedicated “management account” with cross-account roles specifically for Terraform, adhering to the principle of least privilege. We also store remote state in a centralized state account with appropriate access controls.
Question 13: How do you handle large-scale refactoring of resources without downtime?
Answer: Large-scale refactoring requires careful planning and execution. My approach includes:
- State management operations to rename or move resources:
# Move a resource to a module
terraform state mv aws_iam_role.lambda module.lambda_function.aws_iam_role.lambda
# Rename a resource
terraform state mv aws_instance.app aws_instance.application
- Import and adopt existing resources into new configurations:
# Import existing resource into new structure
terraform import module.vpc.aws_subnet.private[1] subnet-0a9fcbce2e2bf8eac
- Targeted applies to control the scope of changes:
terraform apply -target=module.networking -target=module.security
- For high-risk refactorings, I use state manipulation with precise terraform plan verification:
# Extract current state
terraform state pull > current-state.json
# Perform refactoring in code
# Verify changes won't destroy critical resources
terraform plan -out=refactor.plan
terraform show -json refactor.plan | jq '.resource_changes[] | select(.change.actions[] | contains("delete"))'
# Apply with state manipulation if needed
- Progressive changes by splitting refactoring into multiple non-destructive PRs.
In a recent project, we refactored a monolithic Terraform configuration into a modular structure across 200+ resources without any service downtime by combining these techniques with a comprehensive testing strategy.
Question 14: How do you implement dynamic resource creation based on external data sources?
Answer: Dynamic resource creation often requires combining external data sources with Terraform’s for_each
or count
. My implementation typically includes:
# Fetch external data
data "external" "user_config" {
program = ["python", "${path.module}/scripts/get_config.py"]
}
# Parse data
locals {
user_data = jsondecode(data.external.user_config.result.users)
# Transform for use with for_each
users_map = {
for user in local.user_data :
user.username => user
}
}
# Create resources dynamically
resource "aws_iam_user" "team" {
for_each = local.users_map
name = each.key
tags = {
Department = each.value.department
Role = each.value.role
}
}
resource "aws_iam_user_policy_attachment" "user_permissions" {
for_each = local.users_map
user = aws_iam_user.team[each.key].name
policy_arn = "arn:aws:iam::aws:policy/${each.value.policy}"
}
For more complex scenarios, I’ve integrated with external systems using the HTTP provider or custom data sources:
data "http" "active_services" {
url = "https://service-registry.example.com/api/services"
request_headers = {
Authorization = "Bearer ${var.api_token}"
}
}
locals {
services = jsondecode(data.http.active_services.response_body).items
}
# Create load balancer target groups dynamically
resource "aws_lb_target_group" "services" {
for_each = { for svc in local.services : svc.name => svc }
name = each.key
port = each.value.port
protocol = "HTTP"
vpc_id = var.vpc_id
}
This approach allows infrastructure to adapt automatically to changing requirements without manual intervention, while maintaining the declarative nature of Terraform.
Question 15: How do you implement custom providers or extend existing Terraform providers?
Answer: When standard providers can’t meet specific requirements, I use custom providers or provider extensions:
- Creating custom providers involves developing a Go application using the Terraform Plugin Framework:
package main
import (
"github.com/hashicorp/terraform-plugin-sdk/v2/helper/schema"
"github.com/hashicorp/terraform-plugin-sdk/v2/plugin"
)
func main() {
plugin.Serve(&plugin.ServeOpts{
ProviderFunc: Provider,
})
}
func Provider() *schema.Provider {
return &schema.Provider{
ResourcesMap: map[string]*schema.Resource{
"custom_resource": resourceCustomResource(),
},
DataSourcesMap: map[string]*schema.Resource{
"custom_data_source": dataSourceCustomData(),
},
}
}
// Resource and data source implementations...
- Provider wrappers use existing providers with custom pre/post processing:
module "aws_resource_wrapper" {
source = "./modules/resource_wrapper"
resource_config = {
type = "aws_instance"
attributes = {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
}
}
pre_create_hook = "scripts/pre_create.sh"
post_create_hook = "scripts/post_create.sh"
}
- External data sources extend functionality without full provider development:
data "external" "custom_processor" {
program = ["python", "${path.module}/scripts/custom_logic.py"]
query = {
input_param = var.parameter
}
}
resource "aws_instance" "example" {
ami = data.external.custom_processor.result.ami_id
instance_type = data.external.custom_processor.result.instance_type
}
In enterprise environments, we’ve developed custom providers for internal systems where no public provider exists, such as proprietary CMDB systems or custom deployment platforms.
Question 16: How do you implement safe database schema migrations with Terraform?
Answer: Database schema migrations require special handling in Terraform to avoid data loss. My approach includes:
- Separate the database instance provisioning from schema management:
# Terraform manages the database instance
resource "aws_db_instance" "main" {
identifier = "app-database"
allocated_storage = 20
engine = "postgres"
engine_version = "13.4"
instance_class = "db.t3.medium"
# ...
}
# Output connection information
output "db_endpoint" {
value = aws_db_instance.main.endpoint
sensitive = true
}
- Use dedicated schema migration tools triggered by Terraform:
resource "null_resource" "db_migrations" {
triggers = {
db_instance = aws_db_instance.main.id
migration_hash = filemd5("${path.module}/migrations/")
}
provisioner "local-exec" {
command = "PGPASSWORD=${var.db_password} flyway -url=jdbc:postgresql://${aws_db_instance.main.endpoint}:5432/${aws_db_instance.main.name} -user=${var.db_username} -locations=filesystem:${path.module}/migrations migrate"
}
depends_on = [aws_db_instance.main]
}
- For critical production databases, implement blue/green database deployments:
# Create a read replica
resource "aws_db_instance" "replica" {
replicate_source_db = aws_db_instance.main.id
instance_class = "db.t3.medium"
# ...
}
# Apply schema changes to replica
resource "null_resource" "schema_migration" {
# migration commands to replica
}
# Promote replica to primary (handled outside Terraform or with custom logic)
- Implement comprehensive backup procedures before migrations:
resource "null_resource" "pre_migration_backup" {
provisioner "local-exec" {
command = "aws rds create-db-snapshot --db-instance-identifier ${aws_db_instance.main.id} --db-snapshot-identifier pre-migration-$(date +%Y%m%d-%H%M%S)"
}
}
This approach separates concerns appropriately, leveraging Terraform’s strengths for infrastructure while using specialized tools for schema migrations, minimizing risk to data.
Question 17: How do you implement proper Terraform state locking in a team environment?
Answer: State locking prevents concurrent executions from corrupting the state file. In a team environment, I implement:
- Remote backend with native locking:
terraform {
backend "s3" {
bucket = "terraform-states"
key = "myapp/terraform.tfstate"
region = "us-east-1"
dynamodb_table = "terraform-lock"
}
}
- Custom locking mechanisms for backends without native support:
resource "null_resource" "acquire_lock" {
provisioner "local-exec" {
command = "./scripts/acquire_lock.sh ${var.environment}"
}
}
# Terraform resources...
resource "null_resource" "release_lock" {
provisioner "local-exec" {
command = "./scripts/release_lock.sh ${var.environment}"
}
depends_on = [null_resource.acquire_lock, aws_instance.app]
}
- CI/CD integration with queue-based execution:
# GitLab CI example
terraform_apply:
stage: deploy
script:
- terraform init
- terraform apply -auto-approve
resource_group: terraform-${CI_ENVIRONMENT_NAME}
- Force-unlock procedures for emergency situations:
#!/bin/bash
# Script for authorized force-unlock
if [[ $(aws dynamodb get-item --table-name terraform-lock --key '{"LockID":{"S":"myapp/terraform.tfstate"}}' --query 'Item.Info.S' --output text) == *"${LAST_OPERATOR}"* ]]; then
terraform force-unlock -force $LOCK_ID
echo "Lock forcibly removed"
else
echo "Not authorized to remove lock created by another operator"
exit 1
fi
To enhance team workflow, we’ve also implemented pre-commit hooks for Terraform formatting and validation, as well as automated state lock monitoring that alerts if locks persist for too long (potentially indicating a failed run).
Question 18: How do you implement GitOps workflows with Terraform?
Answer: GitOps with Terraform combines infrastructure as code with Git-based workflows. My implementation includes:
- Branch-based environments with automated workflows:
# GitHub Actions workflow
name: Terraform GitOps
on:
push:
branches:
- main
- staging
- development
pull_request:
branches:
- main
jobs:
terraform:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- name: Configure AWS credentials
uses: aws-actions/configure-aws-credentials@v1
with:
role-to-assume: ${{ secrets.AWS_ROLE_ARN }}
aws-region: us-east-1
- name: Setup Terraform
uses: hashicorp/setup-terraform@v2
- name: Determine environment
id: env
run: |
if [[ "${{ github.ref }}" == "refs/heads/main" ]]; then
echo "environment=production" >> $GITHUB_OUTPUT
elif [[ "${{ github.ref }}" == "refs/heads/staging" ]]; then
echo "environment=staging" >> $GITHUB_OUTPUT
else
echo "environment=development" >> $GITHUB_OUTPUT
fi
- name: Terraform Init
run: terraform init -backend-config=${{ steps.env.outputs.environment }}.backend.hcl
- name: Terraform Plan
run: terraform plan -var-file=${{ steps.env.outputs.environment }}.tfvars -out=tfplan
- name: Terraform Apply
if: github.event_name == 'push'
run: terraform apply tfplan
- Pull request automation with automated plan generation:
# Comment generation for PR
resource "github_repository_webhook" "terraform_plan" {
repository = "infrastructure-repo"
configuration {
url = "https://automation.example.com/terraform-plan"
content_type = "json"
insecure_ssl = false
}
events = ["pull_request"]
}
- Drift detection for ensuring state matches Git:
#!/bin/bash
# Run in scheduled pipeline
terraform plan -detailed-exitcode
if [ $? -eq 2 ]; then
# Drift detected
gh issue create --title "Infrastructure drift detected" \
--body "Terraform detected differences between current state and configuration in Git."
fi
- Approval workflows for production changes:
# Atlantis configuration
repos:
- id: /.*/
workflow: production
workflows:
production:
plan:
steps:
- init
- plan
apply:
steps:
- apply
requires:
- approved
This approach ensures all infrastructure changes go through Git, maintaining a clear audit trail and enabling code review processes before infrastructure changes take effect.
Question 19: How do you implement effective Terraform module testing?
Answer: Comprehensive module testing ensures reliability and reusability. My testing strategy includes:
- Unit testing with Terratest:
package test
import (
"testing"
"github.com/gruntwork-io/terratest/modules/terraform"
"github.com/stretchr/testify/assert"
)
func TestVpcModule(t *testing.T) {
terraformOptions := &terraform.Options{
TerraformDir: "../modules/vpc",
Vars: map[string]interface{}{
"cidr_block": "10.0.0.0/16",
"environment": "test",
},
}
defer terraform.Destroy(t, terraformOptions)
terraform.InitAndApply(t, terraformOptions)
vpcId := terraform.Output(t, terraformOptions, "vpc_id")
privateSubnets := terraform.OutputList(t, terraformOptions, "private_subnet_ids")
assert.NotEmpty(t, vpcId)
assert.Equal(t, 3, len(privateSubnets))
}
- Static analysis using multiple tools:
# CI pipeline
terraform_check:
script:
- tflint --recursive
- terraform validate
- checkov -d .
- terraform-docs markdown . > README.md
- Example-based testing with reference implementations:
modules/
vpc/
main.tf
variables.tf
outputs.tf
README.md
examples/
complete/
main.tf # Reference implementation
outputs.tf
terraform.tfvars.example
README.md
- Integration testing in ephemeral environments:
provider "aws" {
region = "us-east-1"
default_tags {
tags = {
Environment = "test"
Temporary = "true"
AutoDestroy = formatdate("YYYY-MM-DD", timeadd(timestamp(), "24h"))
}
}
}
- Compliance testing using OPA (Open Policy Agent):
# policy.rego
package terraform
deny[msg] {
resource := input.resource.aws_instance[name]
not resource.tags.Owner
msg := sprintf("EC2 instance '%v' is missing required Owner tag", [name])
}
By combining these approaches, we ensure modules work correctly in isolation and as part of larger systems, automatically catch issues before deployment, and maintain consistent quality across all infrastructure components.
Question 20: How do you implement effective dependency management between Terraform stacks?
Answer: Managing dependencies between Terraform stacks while maintaining separation of concerns requires careful design:
- Remote state data sources for explicit dependencies:
# In network stack outputs
output "vpc_id" {
value = aws_vpc.main.id
}
output "private_subnet_ids" {
value = aws_subnet.private[*].id
}
# In application stack
data "terraform_remote_state" "network" {
backend = "s3"
config = {
bucket = "terraform-states"
key = "network/terraform.tfstate"
region = "us-east-1"
}
}
resource "aws_instance" "app" {
subnet_id = data.terraform_remote_state.network.outputs.private_subnet_ids[0]
# ...
}
- Dependency inversion with input variables:
# Application module
variable "vpc_id" {
description = "ID of the VPC where resources will be created"
type = string
}
# In root module
module "network" {
source = "./modules/network"
}
module "application" {
source = "./modules/application"
vpc_id = module.network.vpc_id
depends_on = [module.network]
}
- Output stability contracts to prevent breaking changes:
# Versioned outputs
output "vpc_info_v1" {
value = {
id = aws_vpc.main.id
cidr_block = aws_vpc.main.cidr_block
# Additional attributes...
}
}
- Explicit dependency management tools like Terragrunt:
# terragrunt.hcl
terraform {
source = "git::https://example.com/terraform-aws-modules/application.git?ref=v1.0.0"
}
dependency "vpc" {
config_path = "../vpc"
}
dependency "database" {
config_path = "../database"
}
inputs = {
vpc_id = dependency.vpc.outputs.vpc_id
subnet_ids = dependency.vpc.outputs.private_subnets
db_host = dependency.database.outputs.db_endpoint
}
- For enterprise environments, implement asynchronous dependency resolution through CI/CD pipelines:
# CI/CD pipeline stages
stages:
- network
- data_stores
- applications
- monitoring
network_job:
stage: network
# ...
database_job:
stage: data_stores
needs:
- network_job
# ...
These approaches maintain stack independence while ensuring proper deployment order and data flow between components. The key is to create clear contracts between stacks, implement versioning for stability, and automate dependency resolution through tooling.
Conclusion
Mastering Terraform goes beyond basic syntax and into deep understanding of cloud infrastructure, state management, security best practices, and integration patterns. The engineers who can confidently answer these questions demonstrate the experience needed to handle enterprise-scale Infrastructure as Code implementations.
As you prepare for your next Terraform role, focus not just on “how” to implement features, but on solving the complex real-world challenges that come with managing infrastructure at scale.